Making use of machine studying methodology to immediate constructing
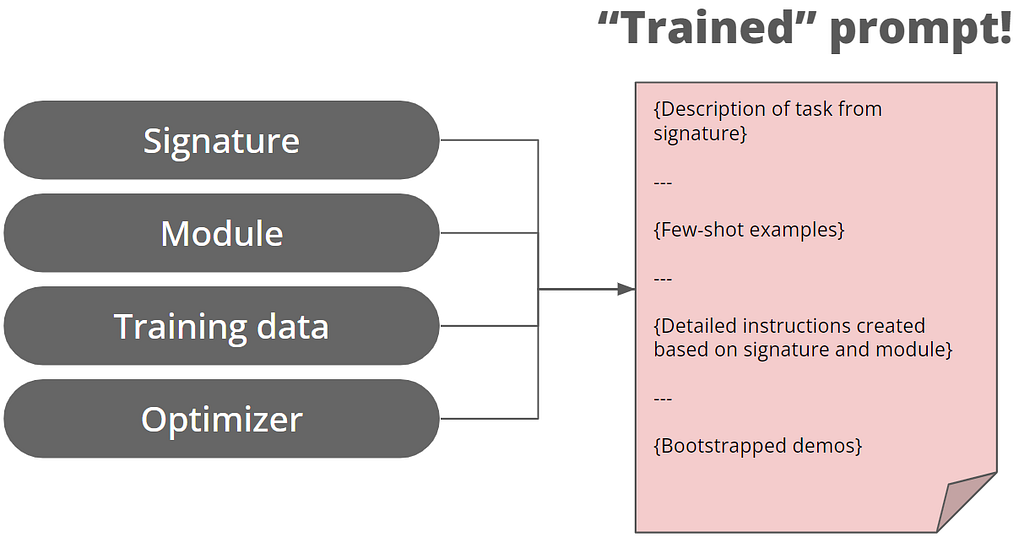
LLMs are grounded in information science, however our strategy to immediate engineering may strike us as unscientific:
- Handbook immediate engineering which doesn’t generalize effectively: LLMs are extremely delicate to how they’re prompted for every job, so we have to handcraft lengthy strings of directions and demonstrations. This requires not solely time-consuming immediate writing course of, however the given string immediate may not generalize to completely different pipelines or throughout completely different LMs, information domains, and even inputs. To cope with a brand new downside we frequently must handcraft a brand new immediate.
- Lack framework to conduct testing: As an alternative of the standard train-test regime in typical information science purposes to choose the mannequin which maximizes a sure metric like AUC, with LLMs we arrive at one of the best immediate through trial and error, typically with out an goal metric to say how effectively our mannequin is performing. Thus irrespective of how we attempt to enhance the immediate, we are able to’t confidently say how dependable our utility is.
To deal with these points, Stanford NLP has printed a paper introducing a brand new strategy with immediate writing: as a substitute of manipulating free-form strings, we generate prompts through modularized programming. The related library, known as DSPy, might be discovered right here.
This text goals to indicate how this “immediate programming” is finished, to go deeper in explaining what’s taking place behind the optimization course of. The code will also be discovered right here.
(Talking of which, you may additionally discover coaxing LLMs to output correctly formatted JSON very unscientific too, I’ve additionally written an article about the way to tackle this with Perform Calling. Test it out !)
Construct Autonomous AI Brokers with Perform Calling
We’ll spend a while to go over the atmosphere preparation. Afterwards, this text is split into 3 sections:
- Primary idea of DSPy: Signature and Module
Primary constructing blocks in DSPy for describing your job, and the immediate method used - Optimizer: Practice our immediate as with machine studying
How DSPy optimizes your immediate with bootstrapping - Full fledged instance: Immediate comparability with LLM
Making use of the rigour of conventional machine studying for immediate testing and choice
We at the moment are able to begin!
Preparation
- Head over to Github to clone my code. The contents in my article might be discovered within the dspy_tutorial Pocket book.
- Please additionally create and activate a digital atmosphere, then pip set up -r necessities.txt to put in the required packages. If you’re on Home windows, please additionally set up Home windows C++ construct instruments that are required for the phoneix library with which we’ll observe how DSPy works
- My code makes use of OpenRouter, which permit us to entry OpenAI API in blocked areas. Please arrange your OPENROUTER_API_KEY as atmosphere variable, and execute the code beneath the “Preparation” block. Alternatively, you should use dspy.OpenAI class straight and outline Open AI API key if it really works for you
Primary idea of DSPy: Signature and Module
They’re the constructing blocks of immediate programming in DSPy. Let’s dive in to see what they’re about!
Signatures: Specification of enter/output
A signature is probably the most elementary constructing block in DSPy’s immediate programming, which is a declarative specification of enter/output habits of a DSPy module. Signatures assist you to inform the LM what it must do, moderately than specify how we should always ask the LM to do it.
Say we need to get hold of the sentiment of a sentence, historically we’d write such immediate:
Given a sentence {the_sentence_itself}, deduce its sentiment.
However in DSPy, we are able to obtain the identical by defining a signature as beneath. At its most simple kind, a signature is so simple as a single string separating the inputs and output with a ->
Observe: Code on this part comprises these referred from DSPy’s documentation of Signatures
# Outline signature
signature = 'sentence -> sentiment'
classify = dspy.Predict(signature)
# Run
sentence = "it's a captivating and infrequently affecting journey."
classify(sentence=sentence).sentiment
--- Output ---
"I'm sorry, however I'm unable to find out the sentiment of the sentence with out extra context or info. For those who present me with extra particulars or particular standards for figuring out sentiment, I might be completely satisfied to help you additional."
The prediction just isn’t a superb one, however for educational function let’s examine what was the issued immediate.
# That is how we inpect the final issued immediate to the LM
lm.inspect_history(n=1)
--- Output ---
Given the fields `sentence`, produce the fields `sentiment`.
---
Observe the next format.
Sentence: ${sentence}
Sentiment: ${sentiment}
---
Sentence: it's a captivating and infrequently affecting journey.
Sentiment: I'm sorry, however I'm unable to find out the sentiment of the sentence with out extra context or info. For those who present me with extra particulars or particular standards for figuring out sentiment, I might be completely satisfied to help you additional.
We will see the above immediate is assembled from the sentence -> sentiment signature. However how did DSPy got here up with the Given the fields… within the immediate?
Inspecting the dspy.Predict() class, we see once we cross to it our signature, the signature will probably be parsed because the signature attribute of the category, and subsequently assembled as a immediate. The directions is a default one hardcoded within the DSPy library.
# Test the variables of the `classify` object,
# which was created by passing the signature to `dspy.Predict()` class
vars(classify)
--- Output ---
{
'signature': StringSignature(sentence -> sentiment
directions='Given the fields `sentence`, produce the fields `sentiment`.'
sentence = Area(annotation=str required=True json_schema_extra={'__dspy_field_type': 'enter', 'prefix': 'Sentence:', 'desc': '${sentence}'})
sentiment = Area(annotation=str required=True json_schema_extra={'__dspy_field_type': 'output', 'prefix': 'Sentiment:', 'desc': '${sentiment}'})
),
'some_other_attributes': 'xxx'}
What if we need to present a extra detailed description of our goal to the LLM, past the essential sentence -> sentiment signature? To take action we have to present a extra verbose signature in type of Class-based DSPy Signatures.
Discover we offer no express instruction as to how the LLM ought to get hold of the sentiment. We’re simply describing the duty at hand, and in addition the anticipated output.
# Outline signature in Class-based kind
class Emotion(dspy.Signature):
# Describe the duty
"""Classify feelings in a sentence."""
sentence = dspy.InputField()
# Including description to the output subject
sentiment = dspy.OutputField(desc="Choices: unhappiness, pleasure, love, anger, worry, shock.")
classify_class_based = dspy.Predict(Emotion)
# Situation prediction
classify_class_based(sentence=sentence).sentiment
--- Output ---
Sentence: It's a captivating and infrequently affecting journey.
Sentiment: pleasure
It’s now outputting a a lot better prediction! Once more we see the descriptions we made when defining the class-based DSPy signatures are assembled right into a immediate.
Classify feelings in a sentence.
---
Observe the next format.
Sentence: ${sentence}
Sentiment: Choices: unhappiness, pleasure, love, anger, worry, shock.
---
Sentence: it's a captivating and infrequently affecting journey.
Sentiment: Sentence: It's a captivating and infrequently affecting journey.
Sentiment: pleasure
This may do for easy duties, however superior purposes may require refined prompting strategies like Chain of Thought or ReAct. In DSPy these are carried out as Modules
Modules: Abstracting prompting strategies
We could also be used to use “prompting strategies” by hardcoding phrases like let’s assume step-by-step in our immediate . In DSPy these prompting strategies are abstracted as Modules. Let’s see beneath for an instance of making use of our class-based signature to the dspy.ChainOfThought module
# Apply the class-based signature to Chain of Thought
classify_cot = dspy.ChainOfThought(Emotion)
# Run
classify_cot(sentence=sentence).sentiment
# Examine immediate
lm.inspect_history(n=1)
--- Output ---
Classify feelings in a sentence.
---
Observe the next format.
Sentence: ${sentence}
Reasoning: Let's assume step-by-step so as to ${produce the sentiment}. We ...
Sentiment: Choices: unhappiness, pleasure, love, anger, worry, shock.
---
Sentence: it's a captivating and infrequently affecting journey.
Reasoning: Let's assume step-by-step so as to Sentence: It's a captivating and infrequently affecting journey.
Reasoning: Let's assume step-by-step so as to decide the sentiment. The usage of the phrases "charming" and "affecting" suggests optimistic feelings related to enjoyment and emotional impression. We will infer that the general tone is optimistic and heartwarming, evoking emotions of pleasure and presumably love.
Sentiment: Pleasure, love
Discover how the “Reasoning: Let’s assume step-by-step…” phrase is added to our immediate, and the standard of our prediction is even higher now.
In accordance with DSPy’s documentation, as of time of writing DSPy offers the next prompting strategies in type of Modules. Discover the dspy.Predict we used within the preliminary instance can be a Module, representing no prompting method!
- dspy.Predict: Primary predictor. Doesn’t modify the signature. Handles the important thing types of studying (i.e., storing the directions and demonstrations and updates to the LM).
- dspy.ChainOfThought: Teaches the LM to assume step-by-step earlier than committing to the signature’s response.
- dspy.ProgramOfThought: Teaches the LM to output code, whose execution outcomes will dictate the response.
- dspy.ReAct: An agent that may use instruments to implement the given signature.
- dspy.MultiChainComparison: Can evaluate a number of outputs from ChainOfThought to provide a last prediction.
It even have some function-style modules:
6. dspy.majority: Can do primary voting to return the most well-liked response from a set of predictions.
You’ll be able to take a look at additional examples in every module’s respective information.
Chaining the modules
However, what about RAG? We will chain the modules collectively to cope with greater issues!
First we outline a retriever, for our instance we use a ColBERT retriever getting info from Wikipedia Abstracts 2017
# Configure retriever
rm = dspy.ColBERTv2(url='http://20.102.90.50:2017/wiki17_abstracts')
dspy.settings.configure(rm = rm)
Then we outline the RAG class inherited from dspy.Module. It wants two strategies:
- The __init__ methodology will merely declare the sub-modules it wants: dspy.Retrieve and dspy.ChainOfThought. The latter is outlined to implement our context, query -> reply signature.
- The ahead methodology will describe the management circulate of answering the query utilizing the modules we have.
Observe: Code on this part is borrowed from DSPy’s introduction pocket book
# Outline a class-based signature
class GenerateAnswer(dspy.Signature):
"""Reply questions with quick factoid solutions."""
context = dspy.InputField(desc="might include related info")
query = dspy.InputField()
reply = dspy.OutputField(desc="typically between 1 and 5 phrases")
# Chain completely different modules collectively to retrieve info from Wikipedia Abstracts 2017, then cross it as context for Chain of Thought to generate a solution
class RAG(dspy.Module):
def __init__(self, num_passages=3):
tremendous().__init__()
self.retrieve = dspy.Retrieve(okay=num_passages)
self.generate_answer = dspy.ChainOfThought(GenerateAnswer)
def ahead(self, query):
context = self.retrieve(query).passages
reply = self.generate_answer(context=context, query=query)
return reply
Then we make use of the category to carry out a RAG
# Initilize our RAG class
rag = RAG()
# Outline a query and cross it into the RAG class
my_question = "When was the primary FIFA World Cup held?"
rag(query=my_question).reply
--- Output ---
'1930'
Inspecting the immediate, we see that 3 passages retrieved from Wikipedia Abstracts 2017 is interpersed as context for Chain of Thought technology
Reply questions with quick factoid solutions.
---
Observe the next format.
Context: might include related info
Query: ${query}
Reasoning: Let's assume step-by-step so as to ${produce the reply}. We ...
Reply: typically between 1 and 5 phrases
---
Context:
[1] «Historical past of the FIFA World Cup | The FIFA World Cup was first held in 1930, when FIFA president Jules Rimet determined to stage a global soccer match. The inaugural version, held in 1930, was contested as a last match of solely 13 groups invited by the group. Since then, the World Cup has skilled successive expansions and format reworking to its present 32-team last match preceded by a two-year qualifying course of, involving over 200 groups from around the globe.»
[2] «1950 FIFA World Cup | The 1950 FIFA World Cup, held in Brazil from 24 June to 16 July 1950, was the fourth FIFA World Cup. It was the primary World Cup since 1938, the deliberate 1942 and 1946 competitions having been cancelled owing to World Battle II. It was received by Uruguay, who had received the inaugural competitors in 1930, clinching the cup by beating the hosts Brazil 2–1 within the deciding match of the four-team last group (this was the one match not determined by a one-match last). It was additionally the primary match the place the trophy was known as the Jules Rimet Cup, to mark the twenty fifth anniversary of Jules Rimet's presidency of FIFA.»
[3] «1970 FIFA World Cup | The 1970 FIFA World Cup was the ninth FIFA World Cup, the quadrennial worldwide soccer championship for males's nationwide groups. Held from 31 Might to 21 June in Mexico, it was the primary World Cup match staged in North America, and the primary held outdoors Europe and South America. Groups representing 75 nations from all six populated continents entered the competitors, and its qualification rounds started in Might 1968. Fourteen groups certified from this course of to hitch host nation Mexico and defending champions England within the sixteen-team last match. El Salvador, Israel, and Morocco made their first appearances on the last stage, and Peru their first since 1930.»
Query: When was the primary FIFA World Cup held?
Reasoning: Let's assume step-by-step so as to Reply: 1930
Reply: 1930
The above examples may not appear a lot. At its most simple utility the DSPy appeared solely doing nothing that may’t be carried out with f-string, nevertheless it truly current a paradigm shift for immediate writing, as this brings modularity to immediate composition!
First we describe our goal with Signature, then we apply completely different prompting strategies with Modules. To check completely different immediate strategies for a given downside, we are able to merely change the modules used and evaluate their outcomes, moderately than hardcoding the “let’s assume step-by-step…” (for Chain of Thought) or “you’ll interleave Thought, Motion, and Commentary steps” (for ReAct) phrases. The good thing about modularity will probably be demonstrated later on this article with a full-fledged instance.
The facility of DSPy just isn’t solely restricted to modularity, it may well additionally optimize our immediate based mostly on coaching samples, and check it systematically. We will probably be exploring this within the subsequent part!
Optimizer: Practice our immediate as with machine studying
On this part we attempt to optimize our immediate for a RAG utility with DSPy.
Taking Chain of Thought for instance, past simply including the “let’s assume step-by-step” phrase, we are able to enhance its efficiency with a number of tweaks:
- Including appropriate examples (aka few-shot studying).
- Moreover, we are able to bootstrap demonstrations of reasoning to show the LMs to use correct reasoning to cope with the duty at hand.
Doing this manually can be extremely time-consuming and may’t generalize to completely different issues, however with DSPy this may be carried out mechanically. Let’s dive in!
Preparation
#1: Loading check information: Like machine studying, to coach our immediate we have to put together our coaching and check datasets. Initially this cell will take round 20 minutes to run.
from dspy.datasets.hotpotqa import HotPotQA
# For demonstration function we'll use a small subset of the HotPotQA dataset, 20 for coaching and testing every
dataset = HotPotQA(train_seed=1, train_size=20, eval_seed=2023, dev_size=20, test_size=0)
trainset = [x.with_inputs('question') for x in dataset.train]
testset = [x.with_inputs('question') for x in dataset.dev]
len(trainset), len(testset)
Inspecting our dataset, which is principally a set of question-and-answer pairs
Instance({'query': 'At My Window was launched by which American singer-songwriter?', 'reply': 'John Townes Van Zandt'}) (input_keys={'query'})
#2 Arrange Phoenix for observability: To facilitate understanding of the optimization course of, we launch Phoenix to watch our DSPy utility, which is a superb software for LLM observability normally! I’ll skip pasting the code right here, however you may execute it within the pocket book.
Observe: If you’re on Home windows, please additionally set up Home windows C++ Construct Instruments right here, which is important for Phoenix
Immediate Optimization
Then we’re able to see what this opimitzation is about! To “prepare” our immediate, we’d like 3 issues:
- A coaching set. We’ll simply use our 20 query–reply examples from trainset.
- A metric for validation. Right here we use the native dspy.consider.answer_exact_match which checks if the anticipated reply precisely matches the fitting reply (questionable however suffice for demonstration). For real-life purposes you may outline your personal analysis standards
- A selected Optimizer (previously teleprompter). The DSPy library contains a variety of optimization methods and you’ll test them out right here. For our instance we use BootstrapFewShot. As an alternative of describing it right here with prolonged description, I’ll reveal it with code subsequently
Now we prepare our immediate.
from dspy.teleprompt import BootstrapFewShot
# Easy optimizer instance. I'm explicitly stating the default values for max_bootstrapped_demos and max_labeled_demos for demonstration functions
optimizer = BootstrapFewShot(metric=dspy.consider.answer_exact_match, max_bootstrapped_demos=4)
# Compile!
compiled_rag = optimizer.compile(RAG(), trainset=trainset)
--- Profitable execution ought to present this output ---
Bootstrapped 4 full traces after n examples in spherical 0
Earlier than utilizing the compiled_rag to reply a query, let’s see what went behind the scene throughout the coaching course of (aka compile). We launch the Phoenix console by visiting http://localhost:6006/ in browser
In my run I’ve made 14 calls utilizing the RAG class, in every of these calls we put up a query to LM to acquire a prediction.
Seek advice from the outcome abstract desk in my pocket book, 4 right solutions are created from these 14 samples, thus reaching our max_bootstrapped_demos parameter and stopping the calls.
However what are the prompts DSPy issued to acquire the bootstrapped demos? Right here’s the immediate for query #14. We will see as DSPy tries to generate one bootstrapped demo, it could randomly add samples from our trainset for few-short studying.
Reply questions with quick factoid solutions.
---
{Pairs of question-and-answer as samples}
---
Observe the next format.
Context: might include related info
Query: ${query}
Reasoning: Let's assume step-by-step so as to ${produce the reply}. We ...
Reply: typically between 1 and 5 phrases
---
Context:
[1] «Eric Davis (baseball) | Eric Keith Davis (born Might 29, 1962) is a former heart fielder for a number of Main League Baseball groups. Davis was 21 years previous when he broke into the massive leagues on Might 19, 1984 with the Cincinnati Reds, the group for which he's most remembered. Blessed with a uncommon mixture of fantastic foot pace and bat pace, Davis turned the primary main league participant to hit at the very least 30 residence runs and steal at the very least 50 bases in the identical season in 1987.»
[2] «Willie Davis (baseball) | William Henry Davis, Jr. (April 15, 1940 – March 9, 2010) was a middle fielder in Main League Baseball who performed most of his profession for the Los Angeles Dodgers. On the finish of his profession he ranked seventh in main league historical past in putouts (5449) and whole possibilities (5719) within the outfield, and third in video games in heart subject (2237). He was ninth in Nationwide League historical past in whole outfield video games (2274), and received Gold Glove Awards from 1971 to 1973. He had 13 seasons of 20 or extra stolen bases, led the NL in triples twice, and retired with the fourth most triples (138) by any main leaguer since 1945. He holds Los Angeles membership information (1958–current) for profession hits (2091), runs (1004), triples (110), at bats (7495), whole bases (3094) and additional base hits (585). His 31-game hitting streak in 1969 stays the longest by a Dodger. At one level throughout the streak, when the group was enjoying at residence, the massive message board at Dodger Stadium quoted a message from a telegram despatched to Davis and the group from Zack Wheat, the group's former file holder, at his residence in Missouri.»
[3] «1992 Los Angeles Dodgers season | The 1992 Los Angeles Dodgers season was a poor one for the group because it completed final within the Western Division of the Nationwide League with a file of 63 wins and 99 losses. Regardless of boasting what was nicknamed the "Outfield of Desires", being manned by Eric Davis, Brett Butler, and Darryl Strawberry, accidents to key gamers and slumps from others contributed to the franchise's worst season since shifting to Los Angeles. Moreover, the Dodgers cancelled 4 residence video games throughout the season because of the L.A. Riots. Regardless of the poor end, the Dodgers had some hope for the longer term as first baseman Eric Karros received the Nationwide League Rookie of the Yr Award, the primary of 5 consecutive Dodger gamers to take action. The 1992 season additionally noticed the Dodgers drop tv station KTTV Ch.11 as their chief broadcaster of Dodger baseball, ending a 34 year-35 consecutive season affiliation with that station. Moreover, it was the primary time the Dodgers misplaced 90 video games in a season since 1944.»
Query: Having the mixture of fantastic foot pace and bat pace helped Eric Davis, create what sort of outfield for the Los Angeles Dodgers?
Reasoning: Let's assume step-by-step so as to Reply: "Outfield of Desires"
Reply: "Outfield of Desires"
Time to place the compiled_rag to check! Right here we elevate a query which was answered wrongly in our abstract desk, and see if we are able to get the fitting reply this time.
compiled_rag(query="Which of those publications was most just lately printed, Who Put the Bomp or Self?")
--- Output ---
Prediction(
rationale='Reply: Self',
reply='Self'
)
We now get the fitting reply!
Once more let’s examine the immediate issued. Discover how the compiled immediate is completely different from those that have been used throughout bootstrapping. Aside from the few-shot examples, bootstrapped Context-Query-Reasoning-Reply demonstrations from right predictions are added to the immediate, enhancing the LM’s functionality.
Reply questions with quick factoid solutions.
---
{Pairs of question-and-answer as samples}
---
Observe the next format.
Context: might include related info
Query: ${query}
Reasoning: Let's assume step-by-step so as to ${produce the reply}. We ...
Reply: typically between 1 and 5 phrases
---
{4 units of Context-Query-Reasoning-Reply demonstrations}
---
Context:
[1] «Who Put the Bomp | Who Put The Bomp was a rock music fanzine edited and printed by Greg Shaw from 1970 to 1979. Its title got here from the hit 1961 doo-wop tune by Barry Mann, "Who Put the Bomp". Later, the title was shortened to "Bomp!"»
[2] «Bompiani | Bompiani is an Italian publishing home based mostly in Milan, Italy. It was based in 1929 by Valentino Bompiani.»
[3] «What Colour is Your Parachute? | What Colour is Your Parachute? by Richard Nelson Bolles is a e book for job-seekers that has been in print since 1970 and has been revised yearly since 1975, generally considerably. Bolles initially self-published the e book (December 1, 1970), nevertheless it has been commercially printed since November 1972 by Ten Velocity Press in Berkeley, California. As of September 28, 2010, the e book is out there in 22 languages, it's utilized in 26 nations around the globe, and over ten million copies have been bought worldwide. It is among the most extremely regarded profession recommendation books in print. Within the newest version of the e book, the creator writes about the way to adapt one's job search to the Internet 2.0 age.»
Query: Which of those publications was most just lately printed, Who Put the Bomp or Self?
Reasoning: Let's assume step-by-step so as to Reply: Self
Reply: Self
So the beneath is principally went behind the scene with BootstrapFewShot throughout compilation:
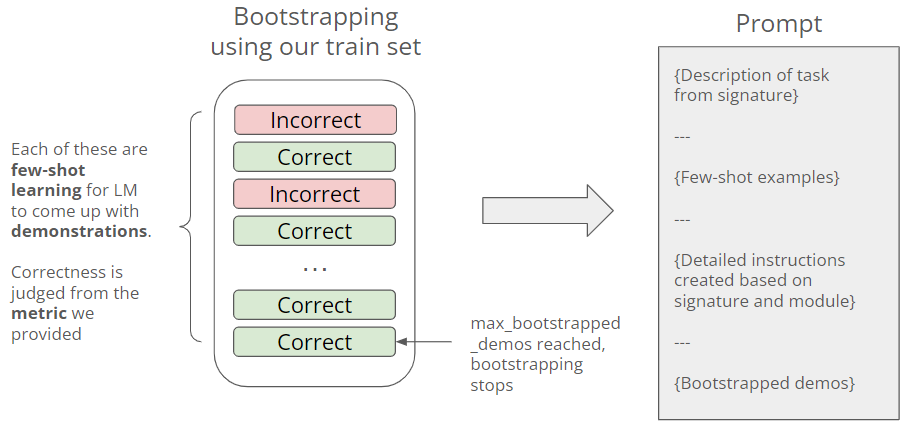
The above instance nonetheless falls wanting what we usually do with machine studying: Even boostrapping possibly helpful, we aren’t but proving it to enhance the standard of the responses.
Ideally, like in conventional machine studying we should always outline a few candidate fashions, see how they carry out in opposition to the check set, and choose the one reaching the best efficiency rating. That is what we’ll do subsequent!
Full fledged instance: Immediate comparability with LLM
The purpose of this instance
On this part, we need to consider what’s the “greatest immediate” (expressed by way of module and optimizer mixture) to carry out a RAG in opposition to the HotpotQA dataset (distributed beneath a CC BY-SA 4.0 License), given the LM we use (GPT 3.5 Turbo).
The Modules beneath analysis are:
- Vanilla: Single-hop RAG to reply a query based mostly on the retrieved context, with out key phrases like “let’s assume step by step”
- COT: Single-hop RAG with Chain of Thought
- ReAct: Single-hop RAG with ReAct prompting
- BasicMultiHop: 2-hop RAG with Chain of Thought
And the Optimizer candidates are:
- None: No extra directions other than the signature
- Labeled few-shot: Merely constructs few-shot examples from offered labeled Q/A pairs
- Bootstrap few-shot: As we demonstrated, self-generate full demonstrations for each stage of our module. Will merely use the generated demonstrations (in the event that they cross the metric) with none additional optimization. For Vanilla it’s simply equal to “Labeled few-shot”
As for analysis metric, we once more use precise match as standards (dspy.consider.metrics.answer_exact_match) in opposition to the check set.
Comparability
Let’s start! First, we outline our modules
# Vanilla
class Vanilla(dspy.Module):
def __init__(self, num_passages=3):
tremendous().__init__()
self.retrieve = dspy.Retrieve(okay=num_passages)
self.generate_answer = dspy.Predict("context, query -> reply")
def ahead(self, query):
context = self.retrieve(query).passages
reply = self.generate_answer(context=context, query=query)
return reply
vanilla = Vanilla()
# COT
class COT(dspy.Module):
def __init__(self, num_passages=3):
tremendous().__init__()
self.retrieve = dspy.Retrieve(okay=num_passages)
self.generate_answer = dspy.ChainOfThought("context, query -> reply")
def ahead(self, query):
context = self.retrieve(query).passages
reply = self.generate_answer(context=context, query=query)
return reply
cot = COT()
# ReAct
react = dspy.ReAct("question-> reply", instruments=[dspy.Retrieve(k=3)], max_iters=5)
# BasicMultiHop
class BasicMultiHop(dspy.Module):
def __init__(self, passages_per_hop=3):
self.retrieve = dspy.Retrieve(okay=passages_per_hop)
self.generate_query = dspy.ChainOfThought("context, question-> search_query")
self.generate_answer = dspy.ChainOfThought("context, question-> reply")
def ahead(self, query):
context = []
for hop in vary(2):
question = self.generate_query(context=context, query=query).search_query
context += self.retrieve(question).passages
return self.generate_answer(context=context, query=query)
multihop = BasicMultiHop(passages_per_hop=3)
Then outline permutations for our mannequin candidates
from dspy.teleprompt import LabeledFewShot, BootstrapFewShot
metric = dspy.consider.metrics.answer_exact_match
modules = {
'vanilla': vanilla,
'cot': cot,
'react': react,
'multihop': multihop,
}
optimizers = {
'none': None,
'labeled_few_shot': LabeledFewShot(),
'bootstrap_few_shot': BootstrapFewShot(metric=metric, max_errors=20),
}
Then I outlined a helper class to facilitate the analysis. The code is a tad bit lengthy so I’m not pasting it right here, nevertheless it may very well be present in my pocket book. What it does is to use every the optimizers in opposition to the modules, compile the immediate, then carry out analysis in opposition to the check set.
We at the moment are prepared to start out the analysis, it could take round 20 minutes to full
# Compile the fashions
ms = ModelSelection(modules=modules, optimizers=optimizers, metric=metric, trainset=trainset)
# Consider them
ms.consider(testset=testset)
Right here’s the analysis outcome. We will see the COT module with BootstrapFewShot optimizer has one of the best efficiency. The scores signify the share of right solutions (judged by precise match) made for the check set.
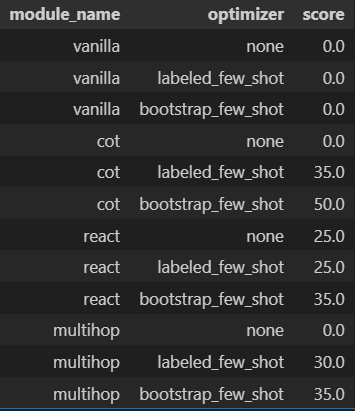
However earlier than we conclude the train, it is likely to be helpful to examine the outcome extra deeply: Multihop with BootstrapFewShot, which supposedly equips with extra related context than COT with BootstrapFewShot, has a worse efficiency. It’s unusual!
Debug and fine-tune our immediate
Now head to the Phoenix Console to see what’s occurring. We choose a random query William Hughes Miller was born in a metropolis with what number of inhabitants ?, and examine how did COT, ReAct, BasicMultiHop with BoostrapFewShot optimizer got here up with their reply. You’ll be able to sort this within the search bar for filter: """William Hughes Miller was born in a metropolis with what number of inhabitants ?""" in enter.worth
These are the solutions offered by the three fashions throughout my run:
- Multihop with BootstrapFewShot: The reply will differ based mostly on the precise metropolis of William Hughes Miller’s birthplace.
- ReAct with BootstrapFewShot: Kosciusko, Mississippi
- COT with BootstrapFewShot: Town of Kosciusko, Mississippi, has a inhabitants of roughly 7,402 inhabitants.
The right reply is 7,402 on the 2010 census. Each ReAct with BootstrapFewShot and COT with BootstrapFewShot offered related solutions, however Multihop with BootstrapFewShot merely failed to supply one.
Checking the execution hint in Phoenix for Multihop with BootstrapFewShot, appears to be like just like the LM fails to know what is predicted for the search_query specified within the signature.
So we revise the signature, and re-run the analysis with the code beneath
# Outline a class-based signature
class GenerateAnswer(dspy.Signature):
"""Reply questions with quick factoid solutions."""
context = dspy.InputField(desc="might include related info")
query = dspy.InputField()
reply = dspy.OutputField(desc="typically between 1 and 5 phrases")
class BasicQA(dspy.Signature):
"""Reply questions with quick factoid solutions."""
query = dspy.InputField()
reply = dspy.OutputField(desc="typically between 1 and 5 phrases")
class FollowupQuery(dspy.Signature):
"""Generate a question which is conducive to answering the query"""
context = dspy.InputField(desc="might include related info")
query = dspy.InputField()
search_query = dspy.OutputField(desc="Decide if the context is sufficient to reply the query, if not sufficient or whether it is clean, generate a search question that might enable you reply the query.")
# Revise the modules with the class-based signatures. You'll find the related code in my pocket book
# To maintain the article concise I'm not pasting it right here.
# Then run the beneath command to re-compile and consider
ms_revised = ModelSelection(modules=modules_revised, optimizers=optimizers, metric=metric, trainset=trainset)
ms_revised.consider(testset=testset)
ms_revised.evaluation_matrix
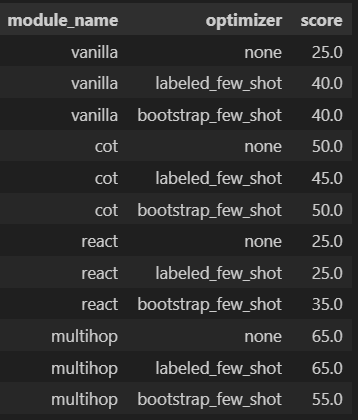
We now see the rating improved throughout all fashions, and Multihop with LabeledFewShot and Multihop with no examples now have one of the best efficiency! This means regardless of DSPy tries to optimize the immediate, there may be nonetheless some immediate engineering concerned by articulating your goal in signature.
The most effective mannequin now produce a precise match for our query!
# The right reply is 7,402
query = """`William Hughes Miller was born in a metropolis with what number of inhabitants ?"""
ms_revised.question_for_model('multihop','labeled_few_shot',query)
--- Output ---
Prediction(
rationale='Reply: 7,402',
reply='7,402'
)
Since one of the best immediate is Multihop with LabeledFewShot, the immediate doesn’t include bootstrapped Context-Query-Reasoning-Reply demonstrations. So bootstrapping might not absolutely result in higher efficiency, we have to show which one is one of the best immediate scientifically.
Reply questions with quick factoid solutions.
---
{Pairs of question-and-answer as samples}
---
Observe the next format.
Context: might include related info
Query: ${query}
Reasoning: Let's assume step-by-step so as to ${produce the reply}. We ...
Reply: typically between 1 and 5 phrases
---
Context:
[1] «William Hughes Miller | William Hughes Miller (born March 16, 1941, Kosciusko, Mississippi) is a professor on the College of California, Berkeley and a number one researcher within the subject of theoretical chemistry.»
[2] «William Herbert Miller, Jr. | William Hubert Miller, Jr. (September 1932 – November 4, 1988), of New York Metropolis, was an aerophilatelist who printed philatelic literature on the topic.»
[3] «William Inexperienced Miller | William Inexperienced Miller (born August 15, 1931 in New York Metropolis, New York), served as america Ambassador to Ukraine beneath Invoice Clinton, from 1993 to 1998.»
[4] «Kosciusko, Mississippi | Kosciusko is a metropolis in Attala County, Mississippi, United States. The inhabitants was 7,402 on the 2010 census. It's the county seat of Attala County.»
[5] «Attala County, Mississippi | Attala County is a county positioned within the U.S. state of Mississippi. As of the 2010 census, the inhabitants was 19,564. Its county seat is Kosciusko. Attala County is called for Atala, a fictional Native American heroine from an early-Nineteenth-century novel of the identical title by François-René de Chateaubriand.»
[6] «Kosciusko Island | Kosciusko Island is an island within the Alexander Archipelago of southeastern Alaska, United States. It lies close to the northwest nook of Prince of Wales Island, simply throughout the El Capitan Passage from the bigger island. The island is close to Mount Francis, Holbrook Mountain, and Tokeen Peak. Kosciusko Island has a land space of 171.585 sq mi (444.403 km²), making it the thirty eighth largest island in america. It had a inhabitants of 52 individuals as of the 2000 census, largely in Edna Bay, its largest group.»
Query: `William Hughes Miller was born in a metropolis with what number of inhabitants ?
Reasoning: Let's assume step-by-step so as to Reply: 7,402
Reply: 7,402
It doesn’t imply Multihop with BootstrapFewShot has a worse efficiency normally nonetheless. Solely that for our job, if we use GPT 3.5 Turbo to bootstrap demonstration (which is likely to be of questionable high quality) and output prediction, then we’d higher do with out the bootstrapping, and hold solely the few-shot examples.
This result in the query: Is it potential to make use of a extra highly effective LM, say GPT 4 Turbo (aka instructor) to generate demonstrations, whereas holding cheaper fashions like GPT 3.5 Turbo (aka scholar) for prediction?
“Instructor” to power-up bootstrapping functionality
The reply is YES as the next cell demonstrates, we’ll use GPT 4 Turbo as instructor.
# Outline the GPT-4 Turbo mannequin
gpt4_turbo = dspy.Databricks(api_key=OPENROUTER_API_KEY,
api_base="https://openrouter.ai/api/v1",
mannequin="openai/gpt-4-turbo")
# Outline new Optimizer which makes use of GPT-4 Turbo as a instructor
optimizers_gpt4_teacher = {
'bootstrap_few_shot': BootstrapFewShot(metric=metric, max_errors=20, teacher_settings=dict(lm=gpt4_turbo)),
}
# Compile the fashions and consider them as earlier than
ms_gpt4_teacher = ModelSelection(modules=modules_revised, optimizers=optimizers_gpt4_teacher, metric=metric, trainset=trainset)
ms_gpt4_teacher.consider(testset=testset)
ms_gpt4_teacher.evaluation_matrix
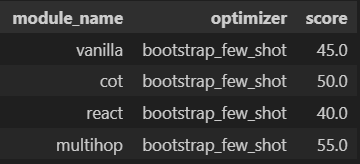
Utilizing GPT-4 Turbo as instructor doesn’t considerably enhance our fashions’ efficiency nonetheless. Nonetheless it’s worthwhile to see its impact to our immediate. Under is the immediate generated simply utilizing GPT 3.5
Reply questions with quick factoid solutions.
---
{Pairs of question-and-answer as samples}
---
Observe the next format.
Context: might include related info
Query: ${query}
Reasoning: Let's assume step-by-step so as to ${produce the reply}. We ...
Reply: typically between 1 and 5 phrases
---
Context:
[1] «Candace Kita | Kita's first position was as a information anchor within the 1991 film "Stealth Hunters". Kita's first recurring tv position was in Fox's "Masked Rider", from 1995 to 1996. She appeared as a collection common lead in all 40 episodes. Kita additionally portrayed a frantic stewardess in a music video directed by Mark Pellington for the British group, Catherine Wheel, titled, "Waydown" in 1995. In 1996, Kita additionally appeared within the movie "Barb Wire" (1996) and visitor starred on "The Wayans Bros.". She additionally visitor starred in "Miriam Teitelbaum: Murder" with "Saturday Evening Dwell" alumni Nora Dunn, "Wall To Wall Information" with Jordan Bridges, "Even Stevens", "Felicity" with Keri Russell, "V.I.P." with Pamela Anderson, "Girlfriends", "The Candy Spot" with Invoice Murray, and "Motion pictures at Our Home". She additionally had recurring roles on the FX spoof, "Son of the Seaside" from 2001 to 2002, ABC-Household's "Dance Fever" and Oxygen Community's "Working with Scissors". Kita additionally appeared within the movies "Little Heroes" (2002) and "Rennie's Touchdown" (2001).»
[2] «Jilly Kitzinger | Jilly Kitzinger is a fictional character within the science fiction collection "Torchwood", portrayed by American actress Lauren Ambrose. The character was promoted as one in every of 5 new primary characters to hitch "Torchwood" in its fourth collection, "" (2011), as a part of a brand new co-production between "Torchwood"' s British community, BBC One, and its American financiers on US premium tv community Starz. Ambrose seems in seven of the ten episodes, and is credited as a "particular visitor star" all through. While response to the serial was combined, Ambrose' portrayal was typically singled out by critics for specific reward and in 2012 she obtained a Saturn Award nomination for Finest Supporting Actress on Tv.»
[3] «Candace Brown | Candace June Brown (born June 15, 1980) is an American actress and comic greatest recognized for her work on exhibits resembling "Gray's Anatomy", "Determined Housewives", "Head Case", The "Wizards Of Waverly Place". In 2011, she joined the visitor solid for "Torchwood"' s fourth collection' "", airing on BBC One in the UK and premium tv community Starz.»
[4] «Candace Kita | Kita's first position was as a information anchor within the 1991 film "Stealth Hunters". Kita's first recurring tv position was in Fox's "Masked Rider", from 1995 to 1996. She appeared as a collection common lead in all 40 episodes. Kita additionally portrayed a frantic stewardess in a music video directed by Mark Pellington for the British group, Catherine Wheel, titled, "Waydown" in 1995. In 1996, Kita additionally appeared within the movie "Barb Wire" (1996) and visitor starred on "The Wayans Bros.". She additionally visitor starred in "Miriam Teitelbaum: Murder" with "Saturday Evening Dwell" alumni Nora Dunn, "Wall To Wall Information" with Jordan Bridges, "Even Stevens", "Felicity" with Keri Russell, "V.I.P." with Pamela Anderson, "Girlfriends", "The Candy Spot" with Invoice Murray, and "Motion pictures at Our Home". She additionally had recurring roles on the FX spoof, "Son of the Seaside" from 2001 to 2002, ABC-Household's "Dance Fever" and Oxygen Community's "Working with Scissors". Kita additionally appeared within the movies "Little Heroes" (2002) and "Rennie's Touchdown" (2001).»
[5] «Kiti Manver | María Isabel Ana Mantecón Vernalte (born 11 Might 1953) higher referred to as Kiti Mánver is a Spanish actress. She has appeared in additional than 100 movies and tv exhibits since 1970. She starred within the 1973 movie "Habla, mudita", which was entered into the twenty third Berlin Worldwide Movie Pageant.»
[6] «Amy Metal | Amy Metal (born Alice Amy Metal; Might 3, 1960) is an American movie and tv actress. She is greatest recognized for her roles as Ginny Area in "Friday the thirteenth Half 2" (1981) and Package Graham in "April Idiot's Day" (1986). She has starred in movies resembling "Uncovered" (1983), "Stroll Like a Man" (1987), "What Ever Occurred to Child Jane? " (1991), and "Tales of Poe" (2014). Metal has had quite a few visitor appearances on a number of tv collection, resembling "Household Ties" (1983), "The A-Crew" (1983), "Quantum Leap" (1990), and "China Seaside" (1991), in addition to a starring position in "The Powers of Matthew Star" (1982–83).»
Query: which American actor was Candace Kita visitor starred with
Reasoning: Let's assume step-by-step so as to Reply: Invoice Murray
Reply: Invoice Murray
---
Context:
[1] «Month-to-month Journal | The Month-to-month Journal (1796–1843) of London started publication in February 1796. Richard Phillips was the writer and a contributor on political points. The editor for the primary ten years was the literary jack-of-all-trades, Dr John Aikin. Different contributors included William Blake, Samuel Taylor Coleridge, George Dyer, Henry Neele and Charles Lamb. The journal additionally printed the earliest fiction of Charles Dickens, the primary of what would change into "Sketches by Boz".»
[2] «Bodega Journal | Bodega Journal is an internet literary journal that releases new points on the primary Monday of each month, that includes tales, poems, essays and interviews from a mixture of rising and established writers. It was based in early spring of 2012 by inventive writing MFA graduates from New York College who had beforehand labored collectively on the "Washington Sq. Evaluation", and continues to be based mostly out of Manhattan and Brooklyn. The inaugural difficulty was printed on September 4, 2012.»
[3] «Who Put the Bomp | Who Put The Bomp was a rock music fanzine edited and printed by Greg Shaw from 1970 to 1979. Its title got here from the hit 1961 doo-wop tune by Barry Mann, "Who Put the Bomp". Later, the title was shortened to "Bomp!"»
[4] «The Most (album) | The Most is the third album launched by straight edge hardcore punk band All the way down to Nothing. It was launched on July 17, 2007.»
[5] «The Most Unbelievable Factor | “The Most Unbelievable Factor" (Danish: "Det Utroligste" ) is a literary fairy story by Danish poet and creator Hans Christian Andersen (1805–1875). The story is a couple of contest to search out probably the most unbelievable factor and the wondrous penalties when the winner is chosen. The story was first printed in an English translation by Horace Scudder, an American correspondent of Andersen's, in america in September 1870 earlier than being printed within the unique Danish in Denmark in October 1870. "The Most Unbelievable Factor" was the primary of Andersen's tales to be printed in Denmark throughout World Battle II. Andersen thought of the story one in every of his greatest.»
[6] «Augusta Triumphans | Augusta Triumphans: or, the Method to Make London the Most Flourishing Metropolis within the Universe by Daniel Defoe was first printed on 16 March 1728. The fictional speaker of this pamphlet, Andrew Moreton, is a person in his sixties who presents strategies for the development of London. Specifically, he fosters the institution of a college, an academy of music, a hospital for foundlings and licensed establishments for the remedy of psychological illnesses. Furthermore, he encourages the introduction of measures to stop ethical corruption and avenue theft.»
Query: Which of those publications was most just lately printed, Who Put the Bomp or Self?
Reasoning: Let's assume step-by-step so as to Reply: Self
Reply: Self
---
Context:
[1] «The Victorians | The Victorians - Their Story In Footage is a 2009 British documentary collection which focuses on Victorian artwork and tradition. The four-part collection is written and introduced by Jeremy Paxman and debuted on BBC One at 9:00pm on Sunday 15 February 2009.»
[2] «What the Victorians Did for Us | What the Victorians Did for Us is a 2001 BBC documentary collection that examines the impression of the Victorian period on fashionable society. It concentrates totally on the scientific and social advances of the period, which bore the Industrial Revolution and set the requirements for well mannered society at this time.»
[3] «The Nice Victorian Assortment | The Nice Victorian Assortment, printed in 1975, is a novel by Northern Irish-Canadian author Brian Moore. Set in Carmel, California, it tells the story of a person who desires that the empty parking zone he can see from his resort window has been reworked by the arrival of a group of priceless Victoriana on show in an unlimited open-air market. When he awakes he finds that he can not distinguish the dream from actuality.»
[4] «Jeremy Paxman | Jeremy Dickson Paxman (born 11 Might 1950) is an English broadcaster, journalist, and creator. He's the query grasp of "College Problem", having succeeded Bamber Gascoigne when the programme was revived in 1994.»
[5] «Jeremy I | Jeremy I used to be king of the Miskito nation, who got here to energy following the dying of his father, Oldman, in 1686 or 1687. in accordance with an English customer, W. M., in 1699, he was about 60 years previous at the moment, making his beginning 12 months about 1639.»
[6] «Jeremy Cheeseman | Jeremy Cheeseman (born June 6, 1990 in Manorville, New York) is a former American skilled soccer participant. Taking part in two seasons for the Dayton Dutch Lions within the USL Skilled Division earlier than retiring because of harm»
Query: The Victorians - Their Story In Footage is a documentary collection written by an creator born in what 12 months?
Reasoning: Let's assume step-by-step so as to Reply: 1950
Reply: 1950
---
Context:
[1] «Tae Kwon Do Instances | Tae Kwon Do Instances is {a magazine} dedicated to the martial artwork of taekwondo, and is printed in america of America. Whereas the title means that it focuses on taekwondo solely, the journal additionally covers different Korean martial arts. "Tae Kwon Do Instances" has printed articles by a variety of authors, together with He-Younger Kimm, Thomas Kurz, Scott Shaw, and Mark Van Schuyver.»
[2] «Scott Shaw (artist) | Scott Shaw (typically spelled Scott Shaw!) is a United States cartoonist and animator, and historian of comics. Amongst Scott's comic-book work is Hanna-Barbera's "The Flintstones" (for Marvel Comics and Harvey Comics), "Captain Carrot and His Superb Zoo Crew" (for DC Comics), and "Simpsons Comics" (for Bongo Comics). He was additionally the primary artist for Archie Comics' "Sonic the Hedgehog" comedian e book collection.»
[3] «Scott Shaw | Scott Shaw (born September 23, 1958) is an American actor, creator, movie director, movie producer, journalist, martial artist, musician, photographer, and professor.»
[4] «Scott Shaw (artist) | Scott Shaw (typically spelled Scott Shaw!) is a United States cartoonist and animator, and historian of comics. Amongst Scott's comic-book work is Hanna-Barbera's "The Flintstones" (for Marvel Comics and Harvey Comics), "Captain Carrot and His Superb Zoo Crew" (for DC Comics), and "Simpsons Comics" (for Bongo Comics). He was additionally the primary artist for Archie Comics' "Sonic the Hedgehog" comedian e book collection.»
[5] «Scott Shaw | Scott Shaw (born September 23, 1958) is an American actor, creator, movie director, movie producer, journalist, martial artist, musician, photographer, and professor.»
[6] «Arnold Shaw (creator) | Arnold Shaw (1909–1989) was a songwriter and music enterprise government, primarily within the subject of music publishing, who's greatest recognized for his complete collection of books on twentieth century American in style music.»
Query: Which journal has printed articles by Scott Shaw, Tae Kwon Do Instances or Southwest Artwork?
Reasoning: Let's assume step-by-step so as to Reply: Tae Kwon Do Instances
Reply: Tae Kwon Do Instances
---
Context:
[1] «William Hughes Miller | William Hughes Miller (born March 16, 1941, Kosciusko, Mississippi) is a professor on the College of California, Berkeley and a number one researcher within the subject of theoretical chemistry.»
[2] «William Herbert Miller, Jr. | William Hubert Miller, Jr. (September 1932 – November 4, 1988), of New York Metropolis, was an aerophilatelist who printed philatelic literature on the topic.»
[3] «William Rickarby Miller | William Rickarby Miller (Might 20, 1818 in Staindrop – July 1893 in New York Metropolis) was an American painter, of the Hudson River College.»
[4] «Kosciusko, Mississippi | Kosciusko is a metropolis in Attala County, Mississippi, United States. The inhabitants was 7,402 on the 2010 census. It's the county seat of Attala County.»
[5] «Attala County, Mississippi | Attala County is a county positioned within the U.S. state of Mississippi. As of the 2010 census, the inhabitants was 19,564. Its county seat is Kosciusko. Attala County is called for Atala, a fictional Native American heroine from an early-Nineteenth-century novel of the identical title by François-René de Chateaubriand.»
[6] «Kosciusko Island | Kosciusko Island is an island within the Alexander Archipelago of southeastern Alaska, United States. It lies close to the northwest nook of Prince of Wales Island, simply throughout the El Capitan Passage from the bigger island. The island is close to Mount Francis, Holbrook Mountain, and Tokeen Peak. Kosciusko Island has a land space of 171.585 sq mi (444.403 km²), making it the thirty eighth largest island in america. It had a inhabitants of 52 individuals as of the 2000 census, largely in Edna Bay, its largest group.»
Query: `William Hughes Miller was born in a metropolis with what number of inhabitants ?
Reasoning: Let's assume step-by-step so as to Reply: 7,402
Reply: 7,402
And right here’s the immediate generated utilizing GPT-4 Turbo as instructor. Discover how the “Reasoning” is a lot better articulated right here!
Reply questions with quick factoid solutions.
---
{Pairs of question-and-answer as samples}
---
Observe the next format.
Context: might include related info
Query: ${query}
Reasoning: Let's assume step-by-step so as to ${produce the reply}. We ...
Reply: typically between 1 and 5 phrases
---
Context:
[1] «Month-to-month Journal | The Month-to-month Journal (1796–1843) of London started publication in February 1796. Richard Phillips was the writer and a contributor on political points. The editor for the primary ten years was the literary jack-of-all-trades, Dr John Aikin. Different contributors included William Blake, Samuel Taylor Coleridge, George Dyer, Henry Neele and Charles Lamb. The journal additionally printed the earliest fiction of Charles Dickens, the primary of what would change into "Sketches by Boz".»
[2] «Who Put the Bomp | Who Put The Bomp was a rock music fanzine edited and printed by Greg Shaw from 1970 to 1979. Its title got here from the hit 1961 doo-wop tune by Barry Mann, "Who Put the Bomp". Later, the title was shortened to "Bomp!"»
[3] «Desktop Publishing Journal | Desktop Publishing journal (ISSN 0884-0873) was based, edited, and printed by Tony Bove and Cheryl Rhodes of TUG/Person Publications, Inc., of Redwood Metropolis, CA. ) . Its first difficulty appeared in October, 1985, and was created and produced on a private pc with desktop publishing software program (PageMaker on a Macintosh), getting ready output on a prototype PostScript-driven typesetting machine from Mergenthaler Linotype Firm. Erik Sandberg-Diment, a columnist at "The New York Instances", tried to purchase the enterprise outright when he noticed an early version.»
[4] «Self (journal) | Self is an American journal for girls that focuses on well being, wellness, magnificence, and magnificence. A part of Condé Nast, Self had a circulation of 1,515,880 and a complete viewers of 5,282,000 readers, in accordance with its company media equipment n 2013. The editor-in-chief is Carolyn Kylstra. "Self" relies within the Condé Nast U.S. headquarters at 1 World Commerce Heart in New York, NY. In February 2017 the journal turned an internet publication.»
[5] «Self-Publishing Evaluation | Self-Publishing Evaluation (or "SPR") is an internet e book evaluation journal for indie authors based in 2008 by American creator Henry Baum.»
[6] «Self-publishing | Self-publishing is the publication of any e book, album or different media by its creator with out the involvement of a longtime writer. A self-published bodily e book is alleged to have been privately printed. The creator is answerable for the whole course of together with, for a e book, the design of the duvet and inside, codecs, value, distribution, advertising and marketing, and public relations. The authors can do all of it themselves or might outsource some or the entire work to firms which supply these companies.»
Query: Which of those publications was most just lately printed, Who Put the Bomp or Self?
Reasoning: Let's assume step-by-step so as to decide which publication was most just lately printed. In accordance with the context, "Who Put the Bomp" was printed from 1970 to 1979. However, "Self" journal turned an internet publication in February 2017 after being a print publication. Due to this fact, "Self" was most just lately printed.
Reply: Self
---
Context:
[1] «The Victorians | The Victorians - Their Story In Footage is a 2009 British documentary collection which focuses on Victorian artwork and tradition. The four-part collection is written and introduced by Jeremy Paxman and debuted on BBC One at 9:00pm on Sunday 15 February 2009.»
[2] «The Nice Victorian Assortment | The Nice Victorian Assortment, printed in 1975, is a novel by Northern Irish-Canadian author Brian Moore. Set in Carmel, California, it tells the story of a person who desires that the empty parking zone he can see from his resort window has been reworked by the arrival of a group of priceless Victoriana on show in an unlimited open-air market. When he awakes he finds that he can not distinguish the dream from actuality.»
[3] «Victorian (comics) | The Victorian is a 25-issue comedian e book collection printed by Penny-Farthing Press and beginning in 1999. The brainchild of creator Trainor Houghton, the collection included a variety of notable script writers and illustrators, together with Len Wein, Glen Orbik and Howard Chaykin.»
[4] «Jeremy Paxman | Jeremy Dickson Paxman (born 11 Might 1950) is an English broadcaster, journalist, and creator. He's the query grasp of "College Problem", having succeeded Bamber Gascoigne when the programme was revived in 1994.»
[5] «Jeremy I | Jeremy I used to be king of the Miskito nation, who got here to energy following the dying of his father, Oldman, in 1686 or 1687. in accordance with an English customer, W. M., in 1699, he was about 60 years previous at the moment, making his beginning 12 months about 1639.»
[6] «Jeremy Cheeseman | Jeremy Cheeseman (born June 6, 1990 in Manorville, New York) is a former American skilled soccer participant. Taking part in two seasons for the Dayton Dutch Lions within the USL Skilled Division earlier than retiring because of harm»
Query: The Victorians - Their Story In Footage is a documentary collection written by an creator born in what 12 months?
Reasoning: Let's assume step-by-step so as to decide the beginning 12 months of the creator who wrote "The Victorians - Their Story In Footage." In accordance with context [4], Jeremy Paxman, an English broadcaster and journalist, wrote and introduced this documentary collection. His beginning 12 months is offered in the identical context.
Reply: 1950
---
Context:
[1] «Tae Kwon Do Instances | Tae Kwon Do Instances is {a magazine} dedicated to the martial artwork of taekwondo, and is printed in america of America. Whereas the title means that it focuses on taekwondo solely, the journal additionally covers different Korean martial arts. "Tae Kwon Do Instances" has printed articles by a variety of authors, together with He-Younger Kimm, Thomas Kurz, Scott Shaw, and Mark Van Schuyver.»
[2] «Kwon Tae-man | Kwon Tae-man (born 1941) was an early Korean hapkido practitioner and a pioneer of the artwork, first in Korea after which in america. He fashioned one of many earliest dojang's for hapkido in america in Torrance, California, and has been featured in lots of journal articles selling the artwork.»
[3] «Scott Shaw (artist) | Scott Shaw (typically spelled Scott Shaw!) is a United States cartoonist and animator, and historian of comics. Amongst Scott's comic-book work is Hanna-Barbera's "The Flintstones" (for Marvel Comics and Harvey Comics), "Captain Carrot and His Superb Zoo Crew" (for DC Comics), and "Simpsons Comics" (for Bongo Comics). He was additionally the primary artist for Archie Comics' "Sonic the Hedgehog" comedian e book collection.»
[4] «Tae Kwon Do Instances | Tae Kwon Do Instances is {a magazine} dedicated to the martial artwork of taekwondo, and is printed in america of America. Whereas the title means that it focuses on taekwondo solely, the journal additionally covers different Korean martial arts. "Tae Kwon Do Instances" has printed articles by a variety of authors, together with He-Younger Kimm, Thomas Kurz, Scott Shaw, and Mark Van Schuyver.»
[5] «Scott Savitt | Scott Savitt is a former international correspondent for The Los Angeles Instances and United Press Worldwide in Beijing. His articles have been printed in The Los Angeles Instances, Washington Publish http://www.washingtonpost.com/wp-dyn/content material/article/2008/04/18/AR2008041802635.html, Wall Avenue Journal, New York Instances, and lots of different publications.»
[6] «Scott Poulson-Bryant | Scott Poulson-Bryant is an award-winning American journalist and creator. One of many co-founding editors of Vibe journal in 1992 (and the editor who gave the journal its title), Poulson-Bryant's journalism, profiles, opinions, and essays have appeared in such publications because the "New York Instances", "the Village Voice", "Rolling Stone", "Spin", "Essence", "Ebony", and "The Supply". He's the creator of "HUNG: A Meditation on the Measure of Black Males in America" (printed by Doubleday Books in 2006) and a novel known as "The VIPs".»
Query: Which journal has printed articles by Scott Shaw, Tae Kwon Do Instances or Southwest Artwork?
Reasoning: Let's assume step-by-step so as to decide which journal printed articles by Scott Shaw. In accordance with the context offered, Scott Shaw has contributed to "Tae Kwon Do Instances," which is talked about in each [1] and [4]. There is no such thing as a point out of Scott Shaw contributing to "Southwest Artwork."
Reply: Tae Kwon Do Instances
---
Context:
[1] «1972 FA Charity Defend | The 1972 FA Charity Defend was contested between Manchester Metropolis and Aston Villa.»
[2] «1968 FA Charity Defend | The 1968 FA Charity Defend was a soccer match performed on 3 August 1968 between Soccer League champions Manchester Metropolis and FA Cup winners West Bromwich Albion. It was the forty sixth Charity Defend match and was performed at Metropolis's residence floor, Maine Street. Manchester Metropolis received 6–1.»
[3] «1973 FA Charity Defend | The 1973 FA Charity Defend was contested between Burnley and Manchester Metropolis in a fixture that passed off at Maine Street.»
[4] «Checklist of Aston Villa F.C. seasons | This can be a record of seasons performed by Aston Villa Soccer Membership in English and European soccer, from 1879 (the 12 months of the membership's first FA Cup entry) to the newest accomplished season. Aston Villa soccer membership was based in March, 1874, by members of the Villa Cross Wesleyan Chapel in Aston. All through the 1870s Aston Villa performed a small quantity of video games. At the least one sport, in opposition to Aston Brook St Mary's was performed with one half beneath Rugby guidelines and the opposite beneath soccer guidelines. Within the Eighties the sport turned extra formalised and in 1888, William McGregor fashioned the Soccer League with 11 different golf equipment.»
[5] «Checklist of Aston Villa F.C. information and statistics | Aston Villa Soccer Membership are an English skilled affiliation soccer membership based mostly in Aston, Birmingham, who presently play within the EFL Championship. The membership was based in 1874 and have performed at their present residence floor, Villa Park, since 1897. Aston Villa have been founding members of the Soccer League in 1888 and the Premier League in 1992. They're one of many oldest and most profitable soccer golf equipment in England, having received the First Division Championship seven occasions and the FA Cup seven occasions. In 1982 the membership turned one in every of solely 5 English golf equipment to win the European Cup.»
[6] «Aston Villa F.C. | Aston Villa Soccer Membership ( ; nicknamed Villa, The Villa, The Villans and The Lions) is knowledgeable soccer membership in Aston, Birmingham, that performs within the Championship, the second stage of English soccer. Based in 1874, they've performed at their present residence floor, Villa Park, since 1897. Aston Villa have been one of many founder members of the Soccer League in 1888 and of the Premier League in 1992.»
Query: In what 12 months was the membership based that performed Manchester Metropolis within the 1972 FA Charity Defend
Reasoning: Let's assume step-by-step so as to decide the founding 12 months of the membership that performed in opposition to Manchester Metropolis within the 1972 FA Charity Defend. In accordance with context [1], the match was contested between Manchester Metropolis and Aston Villa. To search out the founding 12 months of Aston Villa, we seek advice from context [4], which states that Aston Villa Soccer Membership was based in March, 1874.
Reply: 1874
---
Context:
[1] «William Hughes Miller | William Hughes Miller (born March 16, 1941, Kosciusko, Mississippi) is a professor on the College of California, Berkeley and a number one researcher within the subject of theoretical chemistry.»
[2] «William Learn Miller | William Learn Miller (November 23, 1823November 29, 1887) was the twelfth Governor of the State of Arkansas. Born in Batesville, Arkansas; Miller was Arkansas's first native born Governor. Serving two phrases within the turbulent interval after Reconstruction, Miller's four-year administration marked the beginnings of New Departure Democrats in Arkansas. Working on a platform of financial development through reconciliation between whites and freedmen, Miller typically was opposed by members of his personal social gathering throughout the infancy of the Misplaced Trigger ideology. His plans to pay again a big state debt together with the Holford Bonds, valued at $14 million ($ million at this time), have been typically interrupted by racial violence, and his help for public faculties and universities was typically combated by these in his personal social gathering.»
[3] «William "Willie" Armstrong | William Armstrong was born c1804 in Painter Heugh (or Hugh), (which was an previous lane courting from medieval Newcastle, a lane becoming a member of decrease a part of Dean Avenue to the upper a part of Pilgrim Avenue), the title presumably derived from the truth that ships tied up right here within the tidal elements of the Lort Burn (now crammed).»
[4] «Kosciusko, Mississippi | Kosciusko is a metropolis in Attala County, Mississippi, United States. The inhabitants was 7,402 on the 2010 census. It's the county seat of Attala County.»
[5] «Attala County, Mississippi | Attala County is a county positioned within the U.S. state of Mississippi. As of the 2010 census, the inhabitants was 19,564. Its county seat is Kosciusko. Attala County is called for Atala, a fictional Native American heroine from an early-Nineteenth-century novel of the identical title by François-René de Chateaubriand.»
[6] «Kosciusko Island | Kosciusko Island is an island within the Alexander Archipelago of southeastern Alaska, United States. It lies close to the northwest nook of Prince of Wales Island, simply throughout the El Capitan Passage from the bigger island. The island is close to Mount Francis, Holbrook Mountain, and Tokeen Peak. Kosciusko Island has a land space of 171.585 sq mi (444.403 km²), making it the thirty eighth largest island in america. It had a inhabitants of 52 individuals as of the 2000 census, largely in Edna Bay, its largest group.»
Query: `William Hughes Miller was born in a metropolis with what number of inhabitants ?
Reasoning: Let's assume step-by-step so as to Reply: 7,402
Reply: 7,402
Conclusion
Presently we frequently depend on handbook immediate engineering at greatest abstracted as f-string. Additionally, for LM comparability we frequently elevate underspecified questions like “how do completely different LMs evaluate on a sure downside”, borrowed from the Stanford NLP paper’s saying.
However because the above examples reveal, with DSPy’s modular, composable packages and optimizers, we at the moment are geared up to reply towards “how they evaluate on a sure downside with Module X when compiled with Optimizer Y”, which is a well-defined and reproducible run, thus lowering the position of suave immediate building in fashionable AI.
That’s it! Hope you take pleasure in this article.
*Except in any other case famous, all photos are by the creator
Immediate Like a Knowledge Scientist: Auto Immediate Optimization and Testing with DSPy was initially printed in In direction of Knowledge Science on Medium, the place persons are persevering with the dialog by highlighting and responding to this story.