A few years in the past, 4 JavaScript APIs that landed on the backside of consciousness within the State of JavaScript survey. I took an curiosity in these APIs as a result of they’ve a lot potential to be helpful however don’t get the credit score they deserve. Even after a fast search, I used to be amazed at what number of new net APIs have been added to the ECMAScript specification that aren’t getting their dues and with a lack of expertise and browser help in browsers.
That state of affairs generally is a “catch-22”:
Most of those APIs are designed to energy progressive net apps (PWA) and shut the hole between net and native apps. Keep in mind that making a PWA entails extra than simply including a manifest file. Certain, it’s a PWA by definition, nevertheless it features like a bookmark on your own home display screen in follow. In actuality, we’d like a number of APIs to realize a completely native app expertise on the net. And the 4 APIs I’d wish to make clear are a part of that PWA puzzle that brings to the net what we as soon as thought was solely attainable in native apps.
You may see all these APIs in motion on this demo as we go alongside.
1. Display screen Orientation API
The Display screen Orientation API can be utilized to smell out the system’s present orientation. As soon as we all know whether or not a consumer is searching in a portrait or panorama orientation, we are able to use it to improve the UX for cell units by altering the UI accordingly. We will additionally use it to lock the display screen in a sure place, which is helpful for displaying movies and different full-screen components that profit from a wider viewport.
Utilizing the worldwide display screen
object, you’ll be able to entry varied properties the display screen makes use of to render a web page, together with the display screen.orientation
object. It has two properties:
kind
: The present display screen orientation. It may be:"portrait-primary"
,"portrait-secondary"
,"landscape-primary"
, or"landscape-secondary"
.angle
: The present display screen orientation angle. It may be any quantity from 0 to 360 levels, nevertheless it’s usually set in multiples of 90 levels (e.g.,0
,90
,180
, or270
).
On cell units, if the angle
is 0
levels, the kind
is most frequently going to guage to "portrait"
(vertical), however on desktop units, it’s sometimes "panorama"
(horizontal). This makes the kind
property exact for figuring out a tool’s true place.
The display screen.orientation
object additionally has two strategies:
.lock()
: That is an async technique that takes akind
worth as an argument to lock the display screen..unlock()
: This technique unlocks the display screen to its default orientation.
And lastly, display screen.orientation
counts with an "orientationchange"
occasion to know when the orientation has modified.
Browser Help
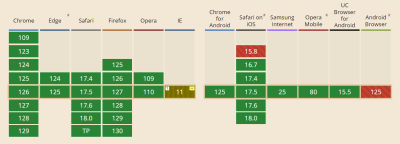
Discovering And Locking Display screen Orientation
Let’s code a brief demo utilizing the Display screen Orientation API to know the system’s orientation and lock it in its present place.
This may be our HTML boilerplate:
Orientation Sort:
Orientation Angle:
On the JavaScript facet, we inject the display screen orientation kind
and angle
properties into our HTML.
let currentOrientationType = doc.querySelector(".orientation-type");
let currentOrientationAngle = doc.querySelector(".orientation-angle");
currentOrientationType.textContent = display screen.orientation.kind;
currentOrientationAngle.textContent = display screen.orientation.angle;
Now, we are able to see the system’s orientation and angle properties. On my laptop computer, they’re "landscape-primary"
and 0°
.
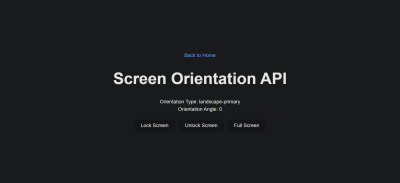
If we hearken to the window’s orientationchange
occasion, we are able to see how the values are up to date every time the display screen rotates.
window.addEventListener("orientationchange", () => {
currentOrientationType.textContent = display screen.orientation.kind;
currentOrientationAngle.textContent = display screen.orientation.angle;
});
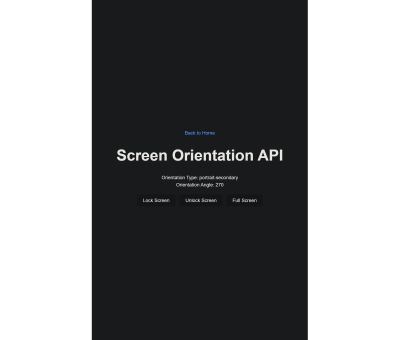
To lock the display screen, we have to first be in full-screen mode, so we are going to use one other extraordinarily helpful characteristic: the Fullscreen API. No person needs a webpage to pop into full-screen mode with out their consent, so we’d like transient activation (i.e., a consumer click on) from a DOM component to work.
The Fullscreen API has two strategies:
Doc.exitFullscreen()
is used from the worldwide doc object,Ingredient.requestFullscreen()
makes the desired component and its descendants go full-screen.
We wish all the web page to be full-screen so we are able to invoke the tactic from the basis component on the doc.documentElement
object:
const fullscreenButton = doc.querySelector(".fullscreen-button");
fullscreenButton.addEventListener("click on", async () => {
// Whether it is already in full-screen, exit to regular view
if (doc.fullscreenElement) {
await doc.exitFullscreen();
} else {
await doc.documentElement.requestFullscreen();
}
});
Subsequent, we are able to lock the display screen in its present orientation:
const lockButton = doc.querySelector(".lock-button");
lockButton.addEventListener("click on", async () => {
attempt {
await display screen.orientation.lock(display screen.orientation.kind);
} catch (error) {
console.error(error);
}
});
And do the alternative with the unlock button:
const unlockButton = doc.querySelector(".unlock-button");
unlockButton.addEventListener("click on", () => {
display screen.orientation.unlock();
});
Can’t We Verify Orientation With a Media Question?
Sure! We will certainly examine web page orientation through the orientation
media characteristic in a CSS media question. Nonetheless, media queries compute the present orientation by checking if the width is “greater than the peak” for panorama or “smaller” for portrait. In contrast,
You could have seen how PWAs like Instagram and X drive the display screen to be in portrait mode even when the native system orientation is unlocked. It is very important discover that this habits isn’t achieved by the Display screen Orientation API, however by setting the orientation
property on the manifest.json
file to the specified orientation kind.
2. Gadget Orientation API
One other API I’d wish to poke at is the Gadget Orientation API. It offers entry to a tool’s gyroscope sensors to learn the system’s orientation in house; one thing used on a regular basis in cell apps, primarily video games. The API makes this occur with a deviceorientation
occasion that triggers every time the system strikes. It has the next properties:
occasion.alpha
: Orientation alongside the Z-axis, starting from 0 to 360 levels.occasion.beta
: Orientation alongside the X-axis, starting from -180 to 180 levels.occasion.gamma
: Orientation alongside the Y-axis, starting from -90 to 90 levels.
Browser Help
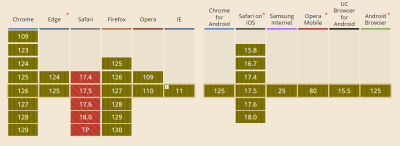
Transferring Parts With Your Gadget
On this case, we are going to make a 3D dice with CSS that may be rotated along with your system! The complete directions I used to make the preliminary CSS dice are credited to David DeSandro and might be present in his introduction to 3D transforms.
See the Pen [Rotate cube [forked]](https://codepen.io/smashingmag/pen/vYwdMNJ) by Dave DeSandro.
You may see uncooked full HTML within the demo, however let’s print it right here for posterity:
Alpha:
Beta:
Gamma:
To maintain this temporary, I received’t clarify the CSS code right here. Simply remember the fact that it offers the mandatory types for the 3D dice, and it may be rotated by all axes utilizing the CSS rotate()
perform.
Now, with JavaScript, we hearken to the window’s deviceorientation
occasion and entry the occasion orientation knowledge:
const currentAlpha = doc.querySelector(".currentAlpha");
const currentBeta = doc.querySelector(".currentBeta");
const currentGamma = doc.querySelector(".currentGamma");
window.addEventListener("deviceorientation", (occasion) => {
currentAlpha.textContent = occasion.alpha;
currentBeta.textContent = occasion.beta;
currentGamma.textContent = occasion.gamma;
});
To see how the info adjustments on a desktop system, we are able to open Chrome’s DevTools and entry the Sensors Panel to emulate a rotating system.
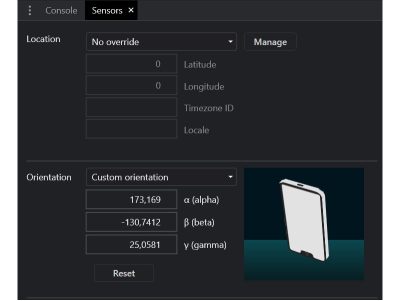
To rotate the dice, we alter its CSS remodel
properties in line with the system orientation knowledge:
const currentAlpha = doc.querySelector(".currentAlpha");
const currentBeta = doc.querySelector(".currentBeta");
const currentGamma = doc.querySelector(".currentGamma");
const dice = doc.querySelector(".dice");
window.addEventListener("deviceorientation", (occasion) => {
currentAlpha.textContent = occasion.alpha;
currentBeta.textContent = occasion.beta;
currentGamma.textContent = occasion.gamma;
dice.type.remodel = `rotateX(${occasion.beta}deg) rotateY(${occasion.gamma}deg) rotateZ(${occasion.alpha}deg)`;
});
That is the consequence:
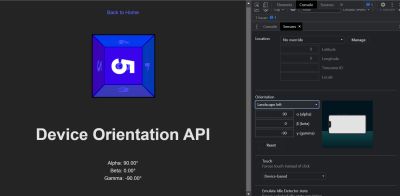
3. Vibration API
Let’s flip our consideration to the Vibration API, which, unsurprisingly, permits entry to a tool’s vibrating mechanism. This turns out to be useful when we have to alert customers with in-app notifications, like when a course of is completed or a message is obtained. That mentioned, we’ve to make use of it sparingly; nobody needs their telephone blowing up with notifications.
There’s only one technique that the Vibration API offers us, and it’s all we’d like: navigator.vibrate()
.
vibrate()
is out there globally from the navigator
object and takes an argument for a way lengthy a vibration lasts in milliseconds. It may be both a quantity or an array of numbers representing a patron of vibrations and pauses.
navigator.vibrate(200); // vibrate 200ms
navigator.vibrate([200, 100, 200]); // vibrate 200ms, wait 100, and vibrate 200ms.
Browser Help
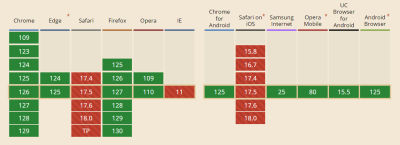
Vibration API Demo
Let’s make a fast demo the place the consumer inputs what number of milliseconds they need their system to vibrate and buttons to start out and cease the vibration, beginning with the markup:
We’ll add an occasion listener for a click on and invoke the vibrate()
technique:
const vibrateButton = doc.querySelector(".vibrate-button");
const millisecondsInput = doc.querySelector("#milliseconds-input");
vibrateButton.addEventListener("click on", () => {
navigator.vibrate(millisecondsInput.worth);
});
To cease vibrating, we override the present vibration with a zero-millisecond vibration.
const stopVibrateButton = doc.querySelector(".stop-vibrate-button");
stopVibrateButton.addEventListener("click on", () => {
navigator.vibrate(0);
});
Previously, it was that solely native apps may hook up with a tool’s “contacts”. However now we’ve the fourth and remaining API I need to have a look at: the Contact Picker API.
The API grants net apps entry to the system’s contact lists. Particularly, we get the contacts.choose()
async technique accessible by the navigator
object, which takes the next two arguments:
properties
: That is an array containing the knowledge we need to fetch from a contact card, e.g.,"identify"
,"handle"
,"electronic mail"
,"tel"
, and"icon"
.choices
: That is an object that may solely comprise thea number of
boolean property to outline whether or not or not the consumer can choose one or a number of contacts at a time.
Browser Help
I’m afraid that browser help is subsequent to zilch on this one, restricted to Chrome Android, Samsung Web, and Android’s native net browser on the time I’m scripting this.
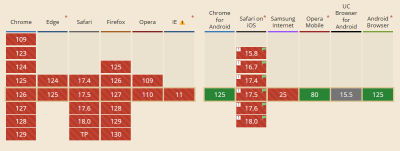
Deciding on Person’s Contacts
We are going to make one other demo to pick and show the consumer’s contacts on the web page. Once more, beginning with the HTML:
Contacts:
Then, in JavaScript, we first assemble our components from the DOM and select which properties we need to decide from the contacts.
const getContactsButton = doc.querySelector(".get-contacts");
const contactList = doc.querySelector(".contact-list");
const props = ["name", "tel", "icon"];
const choices = {a number of: true};
Now, we asynchronously decide the contacts when the consumer clicks the getContactsButton
.
const getContacts = async () => {
attempt {
const contacts = await navigator.contacts.choose(props, choices);
} catch (error) {
console.error(error);
}
};
getContactsButton.addEventListener("click on", getContacts);
Utilizing DOM manipulation, we are able to then append an inventory merchandise to every contact and an icon to the contactList
component.
const appendContacts = (contacts) => {
contacts.forEach(({identify, tel, icon}) => {
const contactElement = doc.createElement("li");
contactElement.innerText = `${identify}: ${tel}`;
contactList.appendChild(contactElement);
});
};
const getContacts = async () => {
attempt {
const contacts = await navigator.contacts.choose(props, choices);
appendContacts(contacts);
} catch (error) {
console.error(error);
}
};
getContactsButton.addEventListener("click on", getContacts);
Appending a picture is somewhat difficult since we might want to convert it right into a URL and append it for every merchandise within the listing.
const getIcon = (icon) => {
if (icon.size > 0) {
const imageUrl = URL.createObjectURL(icon[0]);
const imageElement = doc.createElement("img");
imageElement.src = imageUrl;
return imageElement;
}
};
const appendContacts = (contacts) => {
contacts.forEach(({identify, tel, icon}) => {
const contactElement = doc.createElement("li");
contactElement.innerText = `${identify}: ${tel}`;
contactList.appendChild(contactElement);
const imageElement = getIcon(icon);
contactElement.appendChild(imageElement);
});
};
const getContacts = async () => {
attempt {
const contacts = await navigator.contacts.choose(props, choices);
appendContacts(contacts);
} catch (error) {
console.error(error);
}
};
getContactsButton.addEventListener("click on", getContacts);
And right here’s the result:
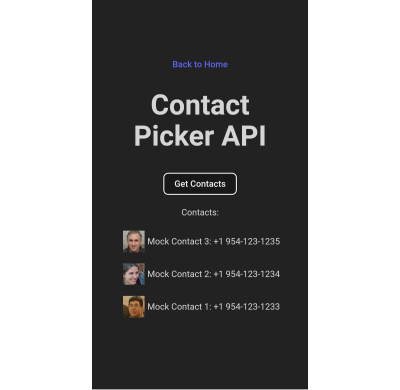
Observe: The Contact Picker API will solely work if the context is safe, i.e., the web page is served over https://
or wss://
URLs.
Conclusion
There we go, 4 net APIs that I imagine would empower us to construct extra helpful and strong PWAs however have slipped beneath the radar for many people. That is, after all, resulting from inconsistent browser help, so I hope this text can convey consciousness to new APIs so we’ve a greater probability to see them in future browser updates.
Aren’t they attention-grabbing? We noticed how a lot management we’ve with the orientation of a tool and its display screen in addition to the extent of entry we get to entry a tool’s {hardware} options, i.e. vibration, and knowledge from different apps to make use of in our personal UI.
However as I mentioned a lot earlier, there’s a kind of infinite loop the place a lack of expertise begets an absence of browser help. So, whereas the 4 APIs we coated are tremendous attention-grabbing, your mileage will inevitably differ relating to utilizing them in a manufacturing surroundings. Please tread cautiously and consult with Caniuse for the newest help info, or examine in your personal units utilizing WebAPI Verify.

(gg, yk)